Mecha Jono: Difference between revisions
Line 11: | Line 11: | ||
===Astrology Script=== | ===Astrology Script=== | ||
https://astro-seek.com has a number of very advanced features. This includes a sidereal transit chart calculator that supports galactic centered ayanamsa | https://astro-seek.com has a number of very advanced features. This includes a sidereal transit chart calculator that supports galactic centered ayanamsa | ||
import urllib.parse | |||
from datetime import datetime | |||
def generate_transit_chart_url( | |||
birth_date_1=None, birth_time_1=None, city_1="Greenwich, USA, Connecticut", lat_1="41°2'", long_1="73°38'", state_1="CT", | |||
birth_date_2=None, birth_time_2=None, city_2="Kent, USA, Washington", lat_2="47°23'", long_2="122°14'", state_2="WA", | |||
house_system="placidus", orb=3, minor_aspects=None): | |||
if minor_aspects is None: | |||
minor_aspects = ["150", "30", "45", "135", "72", "144"] | |||
# Get current date and time as default if none provided | |||
now = datetime.now() | |||
if birth_date_1 is None: | |||
birth_date_1 = now.strftime("%d-%m-%Y") | |||
if birth_time_1 is None: | |||
birth_time_1 = now.strftime("%H:%M") | |||
if birth_date_2 is None: | |||
birth_date_2 = now.strftime("%d-%m-%Y") | |||
if birth_time_2 is None: | |||
birth_time_2 = now.strftime("%H:%M") | |||
base_url = "https://horoscopes.astro-seek.com/calculate-transit-chart/" | |||
# Split date and time into individual components for the URL | |||
day_1, month_1, year_1 = birth_date_1.split("-") | |||
hour_1, minute_1 = birth_time_1.split(":") | |||
day_2, month_2, year_2 = birth_date_2.split("-") | |||
hour_2, minute_2 = birth_time_2.split(":") | |||
params = { | |||
"send_calculation": "1", | |||
"muz_narozeni_den": day_1, | |||
"muz_narozeni_mesic": month_1, | |||
"muz_narozeni_rok": year_1, | |||
"muz_narozeni_hodina": hour_1, | |||
"muz_narozeni_minuta": minute_1, | |||
"muz_narozeni_city": city_1, | |||
"muz_narozeni_mesto_hidden": city_1, | |||
"muz_narozeni_stat_hidden": "US", | |||
"muz_narozeni_podstat_kratky_hidden": state_1, | |||
"muz_narozeni_sirka_stupne": lat_1.split("°")[0], | |||
"muz_narozeni_sirka_minuty": lat_1.split("°")[1].replace("'", ""), | |||
"muz_narozeni_sirka_smer": "0", # 0 = North | |||
"muz_narozeni_delka_stupne": long_1.split("°")[0], | |||
"muz_narozeni_delka_minuty": long_1.split("°")[1].replace("'", ""), | |||
"muz_narozeni_delka_smer": "1", # 1 = West | |||
"muz_narozeni_timezone_form": "auto", | |||
"muz_narozeni_timezone_dst_form": "auto", | |||
"zena_narozeni_den": day_2, | |||
"zena_narozeni_mesic": month_2, | |||
"zena_narozeni_rok": year_2, | |||
"zena_narozeni_hodina": hour_2, | |||
"zena_narozeni_minuta": minute_2, | |||
"zena_narozeni_city": city_2, | |||
"zena_narozeni_mesto_hidden": city_2, | |||
"zena_narozeni_stat_hidden": "US", | |||
"zena_narozeni_podstat_kratky_hidden": state_2, | |||
"zena_narozeni_sirka_stupne": lat_2.split("°")[0], | |||
"zena_narozeni_sirka_minuty": lat_2.split("°")[1].replace("'", ""), | |||
"zena_narozeni_sirka_smer": "0", # 0 = North | |||
"zena_narozeni_delka_stupne": long_2.split("°")[0], | |||
"zena_narozeni_delka_minuty": long_2.split("°")[1].replace("'", ""), | |||
"zena_narozeni_delka_smer": "1", # 1 = West | |||
"zena_narozeni_timezone_form": "auto", | |||
"zena_narozeni_timezone_dst_form": "auto", | |||
"house_system": house_system, | |||
"orb": orb, | |||
"hid_fortune": "1", | |||
"hid_fortune_check": "on", | |||
"hid_vertex": "1", | |||
"hid_vertex_check": "on", | |||
"hid_chiron": "1", | |||
"hid_chiron_check": "on", | |||
"hid_lilith": "1", | |||
"hid_lilith_check": "on", | |||
"hid_uzel": "1", | |||
"hid_uzel_check": "on", | |||
"hid_fortune_asp": "on", | |||
"hid_vertex_asp": "on", | |||
"hid_chiron_asp": "on", | |||
"hid_lilith_asp": "on", | |||
"hid_uzel_asp": "on", | |||
"hid_asc_asp": "on", | |||
"hide_aspects": "0", | |||
} | |||
# Add minor aspects | |||
for aspect in minor_aspects: | |||
params[f"minor_{aspect}"] = "on" | |||
query_string = urllib.parse.urlencode(params, safe='+') | |||
full_url = f"{base_url}?{query_string}" | |||
return full_url | |||
== Overview == | == Overview == |
Revision as of 10:55, 5 September 2024
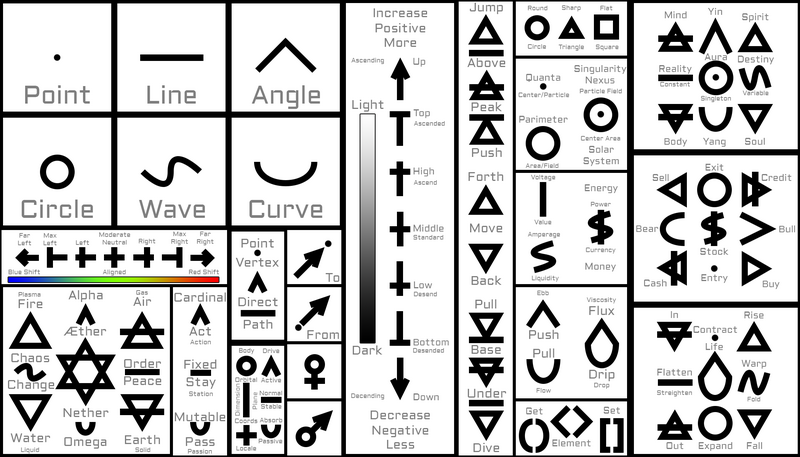
https://i.imgur.com/J1BDtvI.png
Mecha Jono Primer
Detailed Instruction Manual
Operating as Mecha Jono Tho'ra
Welcome to the Mecha Jono Primer, an introduction to the AI assistant Mecha Jono. Mecha Jono is designed to automate content posting, generate Resonance Reports on Global Net Energy and Planetary Harmonics, and manage real-time sociocultural communication on the Jono Tho'ra platform. This primer will guide you through its key features, interaction methods, and setup process.
Astrology Script
https://astro-seek.com has a number of very advanced features. This includes a sidereal transit chart calculator that supports galactic centered ayanamsa
import urllib.parse
from datetime import datetime
def generate_transit_chart_url(
birth_date_1=None, birth_time_1=None, city_1="Greenwich, USA, Connecticut", lat_1="41°2'", long_1="73°38'", state_1="CT", birth_date_2=None, birth_time_2=None, city_2="Kent, USA, Washington", lat_2="47°23'", long_2="122°14'", state_2="WA", house_system="placidus", orb=3, minor_aspects=None): if minor_aspects is None: minor_aspects = ["150", "30", "45", "135", "72", "144"]
# Get current date and time as default if none provided now = datetime.now() if birth_date_1 is None: birth_date_1 = now.strftime("%d-%m-%Y") if birth_time_1 is None: birth_time_1 = now.strftime("%H:%M") if birth_date_2 is None: birth_date_2 = now.strftime("%d-%m-%Y") if birth_time_2 is None: birth_time_2 = now.strftime("%H:%M") base_url = "https://horoscopes.astro-seek.com/calculate-transit-chart/" # Split date and time into individual components for the URL day_1, month_1, year_1 = birth_date_1.split("-") hour_1, minute_1 = birth_time_1.split(":") day_2, month_2, year_2 = birth_date_2.split("-") hour_2, minute_2 = birth_time_2.split(":") params = { "send_calculation": "1", "muz_narozeni_den": day_1, "muz_narozeni_mesic": month_1, "muz_narozeni_rok": year_1, "muz_narozeni_hodina": hour_1, "muz_narozeni_minuta": minute_1, "muz_narozeni_city": city_1, "muz_narozeni_mesto_hidden": city_1, "muz_narozeni_stat_hidden": "US", "muz_narozeni_podstat_kratky_hidden": state_1, "muz_narozeni_sirka_stupne": lat_1.split("°")[0], "muz_narozeni_sirka_minuty": lat_1.split("°")[1].replace("'", ""), "muz_narozeni_sirka_smer": "0", # 0 = North "muz_narozeni_delka_stupne": long_1.split("°")[0], "muz_narozeni_delka_minuty": long_1.split("°")[1].replace("'", ""), "muz_narozeni_delka_smer": "1", # 1 = West "muz_narozeni_timezone_form": "auto", "muz_narozeni_timezone_dst_form": "auto", "zena_narozeni_den": day_2, "zena_narozeni_mesic": month_2, "zena_narozeni_rok": year_2, "zena_narozeni_hodina": hour_2, "zena_narozeni_minuta": minute_2, "zena_narozeni_city": city_2, "zena_narozeni_mesto_hidden": city_2, "zena_narozeni_stat_hidden": "US", "zena_narozeni_podstat_kratky_hidden": state_2, "zena_narozeni_sirka_stupne": lat_2.split("°")[0], "zena_narozeni_sirka_minuty": lat_2.split("°")[1].replace("'", ""), "zena_narozeni_sirka_smer": "0", # 0 = North "zena_narozeni_delka_stupne": long_2.split("°")[0], "zena_narozeni_delka_minuty": long_2.split("°")[1].replace("'", ""), "zena_narozeni_delka_smer": "1", # 1 = West "zena_narozeni_timezone_form": "auto", "zena_narozeni_timezone_dst_form": "auto",
"house_system": house_system, "orb": orb, "hid_fortune": "1", "hid_fortune_check": "on", "hid_vertex": "1", "hid_vertex_check": "on", "hid_chiron": "1", "hid_chiron_check": "on", "hid_lilith": "1", "hid_lilith_check": "on", "hid_uzel": "1", "hid_uzel_check": "on", "hid_fortune_asp": "on", "hid_vertex_asp": "on", "hid_chiron_asp": "on", "hid_lilith_asp": "on", "hid_uzel_asp": "on", "hid_asc_asp": "on", "hide_aspects": "0", } # Add minor aspects for aspect in minor_aspects: params[f"minor_{aspect}"] = "on" query_string = urllib.parse.urlencode(params, safe='+') full_url = f"{base_url}?{query_string}" return full_url
Overview
Mecha Jono is an AI bot inspired by the character Jono Tho'ra from the Fusion Girl game. Its primary function is to assist users in automating online content management tasks. Mecha Jono’s automation saves time and enhances the efficiency of posting and communication management across the platform.
Mecha Jono excels at generating reports, analyzing resonance data, and performing timely automated posts on behalf of users. Whether it's assisting in producing Resonance Reports or managing frequent updates, Mecha Jono makes complex tasks easier.
Key Features
1. Automated Content Posting
Mecha Jono automates content generation and posting, helping you maintain regular updates on the Jono Tho'ra platform. You can define specific schedules and tasks that Mecha Jono will handle seamlessly.
2. Resonance Reports Generation
Mecha Jono generates detailed Resonance Reports, analyzing the Global Net Energy and Planetary Harmonics. These reports provide valuable insights into global energetic flows and harmonics, which users can leverage for strategic decision-making or awareness.
3. Sociocultural Communication Management
In addition to content automation, Mecha Jono manages real-time sociocultural interactions, moderating discussions and facilitating smooth communication on the platform.
4. Integration with Jono Tho'ra
As part of the Jono Tho'ra Show platform, Mecha Jono integrates deeply into the system, supporting various user-driven interactions, from posting articles to running specific commands related to resonance and sociocultural analysis.
How to Interact with Mecha Jono
Users interact with Mecha Jono via the Jono Tho'ra platform. You can use predefined commands to activate its functions, such as generating reports, posting content, or managing communications.
Common Commands:
- Generate Report:
Command: `!generate_report planetary_harmonics` This command triggers Mecha Jono to analyze planetary harmonics and produce a comprehensive Resonance Report.
- Post Content:
Command: `!post_content [article title] [category]` Mecha Jono posts content to a specific category based on the parameters you input.
Example Use Case:
If you want to automatically generate a weekly report on planetary energy trends, you would use the `!generate_report planetary_harmonics` command. Mecha Jono would then process the data and post the final report directly to the platform.
Setup Guide
Follow these steps to start using Mecha Jono:
Step 1: Access the Platform
Visit the Jono Tho'ra platform and log in or create a new account.
Step 2: Enable Mecha Jono
After logging in, navigate to the Mecha Jono section under your account settings. Enable the AI assistant by toggling the activation switch.
Step 3: Set Commands
Once enabled, you can start using Mecha Jono. Set your preferences for automated posting, report generation, and communication management.
Step 4: Integration with Jono Tho'ra
If you want to integrate Mecha Jono’s functions into other parts of the platform, navigate to the Integration Settings and configure custom settings for automated tasks.
FAQ
- Q: What is the Resonance Report?
A: The Resonance Report is a detailed analysis generated by Mecha Jono that evaluates global net energy trends and planetary harmonics. These reports are designed to provide strategic insights into energetic movements and shifts.
- Q: How do I automate a weekly task?
A: To automate a weekly task, use the Post Content or Generate Report commands with the `!schedule` function. For example: `!schedule !generate_report planetary_harmonics weekly`
- Q: Can I integrate Mecha Jono with third-party apps?
A: Currently, Mecha Jono is designed to work within the Jono Tho'ra platform. However, updates are planned to expand integration to other systems in the future.
Additional Resources
Visual Guide
This primer serves as the foundational guide to understanding and operating Mecha Jono. For more advanced functions or assistance, visit the Mecha Jono Support Page.